Carousel
A jQuery plugin that creates a carousel for cycling through elements. Uses CSS transitions to perform the animation.
The carousel comes in two modes: Slide and Fade, both animated via CSS3 transitions. On browsers that do not support transitions they simply change without special effects.
On devices that support touch, grouped items will iterate though their collection via swiping LEFT, and RIGHT
Comes with a markup API that allows running the plugin without writing a single line of JavaScript. This is Responsive's first class API and should be your first consideration when using a plugin.
Examples
Slide Mode
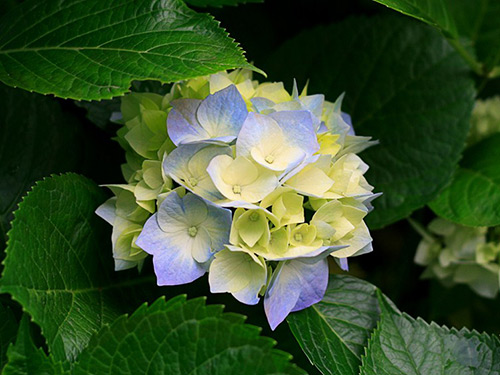
First Thumbnail label
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor.
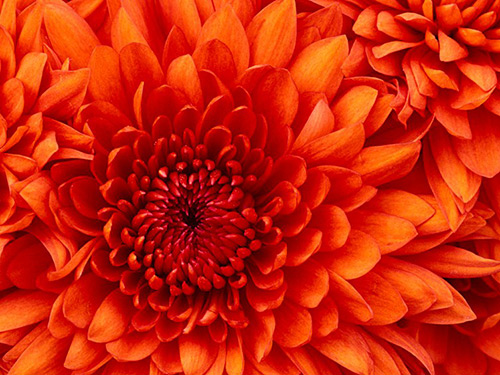
Second Thumbnail label
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor.
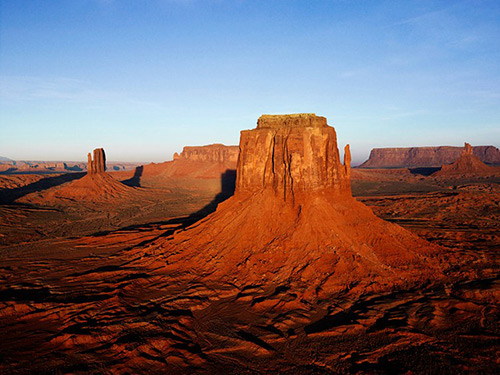
Third Thumbnail label
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor.
Fade Mode
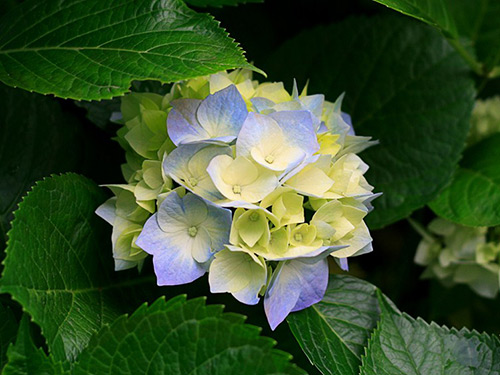
First Thumbnail label
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor.
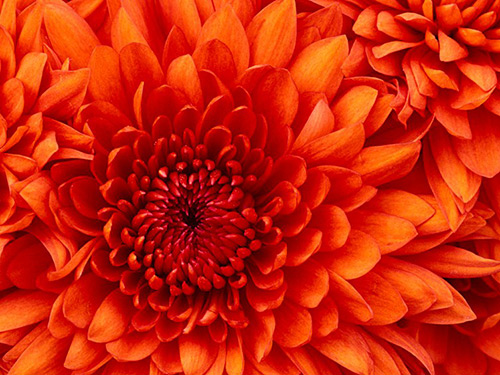
Second Thumbnail label
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor.
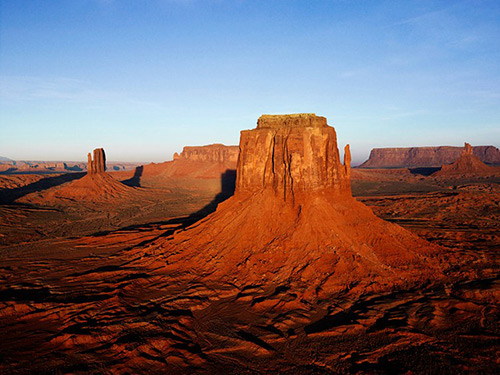
Third Thumbnail label
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor.
Replace image src
attributes with data-src
to allow lazy loading of images.
Markup
<div id="carousel-2" class="carousel" data-carousel-mode="fade" data-carousel-interval="5000">
<!-- Carousel Indicators-->
<ol>
<li data-carousel-target="#carousel-2" data-carousel-slide-to="0" class="active"></li>
<li data-carousel-target="#carousel-2" data-carousel-slide-to="1"></li>
<li data-carousel-target="#carousel-2" data-carousel-slide-to="2"></li>
</ol>
<!-- Carousel Items-->
<figure class="carousel-active">
<img src="../test-assets/img/hydrangeas.jpg" alt="hydrangeas">
<figcaption>
<h4>First Thumbnail label</h4>
<p>Lorem ipsum dolor sit amet, consectetur ...</p>
</figcaption>
</figure>
<figure>
<img src="../test-assets/img/chrysanthemum.jpg" alt="chrysanthemum">
<figcaption>
<h4>Second Thumbnail label</h4>
<p>Lorem ipsum dolor sit amet, consectetur ...</p>
</figcaption>
</figure>
<figure>
<img src="../test-assets/img/desert.jpg" alt="desert">
<figcaption>
<h4>Third Thumbnail label</h4>
<p>Lorem ipsum dolor sit amet, consectetur ...</p>
</figcaption>
</figure>
<!-- Carousel Controls-->
<a class="left carousel-control" href="#carousel-2" data-carousel-slide="prev"><</a>
<a class="right carousel-control" href="#carousel-2" data-carousel-slide="next">></a>
</div>
Methods
The carousel exposes the following method signatures.
- .carousel(options)
-
Initialises the plugin with an optional options
object
Name Type Description Default Value mode string A string containing either the values fade or slide. slide interval number The time in milliseconds between carousel transitions. 5000 pause string The event to bind the pause behaviour to hover wrap boolean Whether the carousel items should wrap true enabletouch boolean Whether the carousel responds to touch events true lazyLoadImages boolean Whether to lazily load any images on page load. true lazyOnDemand boolean Whether to lazily load any images on demand when sliding. Overwrites lazyLoadImages true - .carousel("cycle")
- Cycles through the carousel instance items in a logical order.
- .carousel("pause")
- Triggers the pause event of the carousel instance stopping it cycling.
- .carousel("prev")
- Cycles the carousel instance to the previous item.
- .carousel("next")
- Cycles the carousel instance to the next item.
- .carousel(number)
- Cycles the carousel to a particular item with at the given index (zero based).
Events
The carousel exposes the following events allowing the developer to tap into its behaviour.
- slide.r.carousel
-
This event is fired immediately when the plugins
slide
instance method is invoked. The event data contains two custom properties:- relatedTarget
- The carousel item that will be next in the logical order.
- direction
- The direction in which the carousel is transitioning.
- slid.r.carousel
-
This event is fired when the plugins
slid
instance method is invoked once the carousel has completed sliding. The event data contains two custom properties:- relatedTarget
- The carousel item that will be next in the logical order.
- direction
- The direction in which the carousel is transitioning.
Data API
The carousel's default behaviour is bound using a markup API that allows running the plugin without writing a single line of JavaScript. If you need to override this behaviour the following code will allow you to do so.
// Override the default loading behaviour
$(document).off("ready.r.carousel")
.on("ready.r.carousel", function () {
// Your custom behaviour...
});
// Override the default carousel control click behaviour
$("YOUR_SELECTOR").off("click.r.carousel")
.on("click.r.carousel", "[data-carousel-slide], [data-carousel-slide-to]", function (event) {
// Your custom behaviour...
});
// Bind to the slide event.
$("YOUR_SELECTOR").on("slide.r.carousel", function(event) {
// Your custom behaviour...
});
// Bind to the slid event.
$("YOUR_SELECTOR").on("slid.r.carousel", function(event) {
// Your custom behaviour...
});