Carousel
A jQuery plugin that creates a carousel for cycling through elements. Uses CSS transitions to perform the animation.
Comes with a markup API that allows running the plugin without writing a single line of JavaScript. This is Responsive's first class API and should be your first consideration when using a plugin.
The carousel comes in two modes: Slide and Fade , both animated via CSS3 transitions. On browsers that do not support transitions they simply change without special effects.
Slide Mode
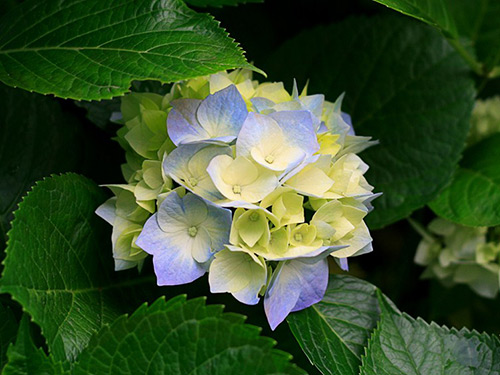
First Thumbnail label
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor.
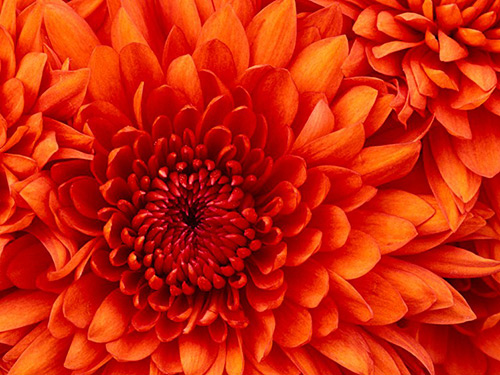
Second Thumbnail label
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor.
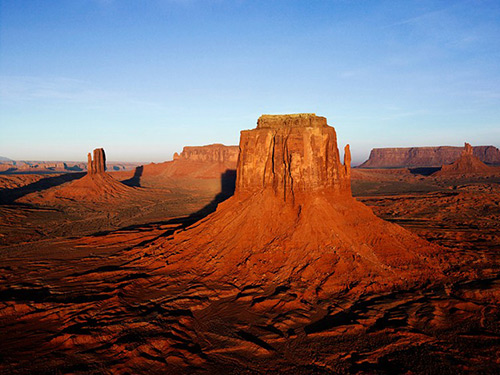
Third Thumbnail label
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor.
Fade Mode
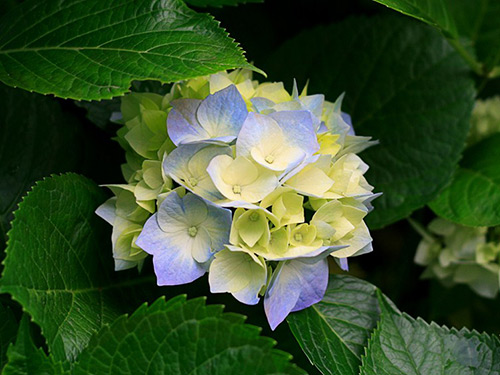
First Thumbnail label
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor.
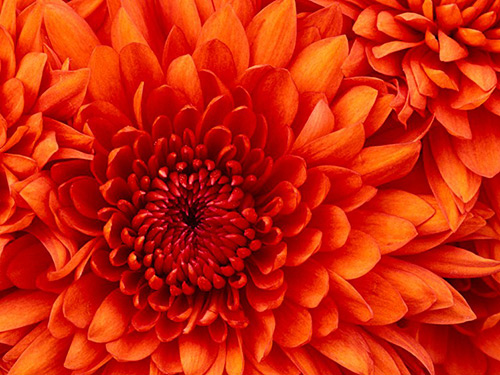
Second Thumbnail label
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor.
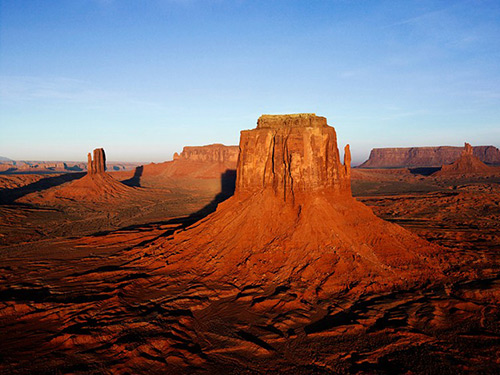
Third Thumbnail label
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor.
If running the plugin in fade mode without using the markup API, add the class .carousel-fade
to the plugin to get the desired style behaviour.
The Markup
<div class="carousel" data-carousel-mode="fade" data-carousel-interval="5000">
<!-- Carousel items -->
<div class="carousel-inner">
<div class="carousel-item carousel-active">...</div>
<div class="carousel-item">...</div>
<div class="carousel-item">...</div>
</div>
<!-- Carousel navigation -->
<a class="carousel-control left" href="#" data-carousel-slide="prev">‹</a>
<a class="carousel-control right" href="#" data-carousel-slide="next">›</a>
</div>
Methods
The carousel exposes the following method signatures.
- .carousel(options)
-
Initialises the plugin with an optional options
object
- options
-
Contains parameters to pass to the plugin.
- mode
- A string containing either the values fade or slide.
- interval
- The time in milliseconds between carousel transitions.
- pause
- The event to bind the pause behaviour to - defaults to hover.
- .carousel("cycle")
- Cycles through the carousel instance items in a logical order.
- .carousel("pause")
- Triggers the pause event of the carousel instance stopping it cycling.
- .carousel("prev")
- Cycles the carousel instance to the previous item.
- .carousel("next")
- Cycles the carousel instance to the next item.
- .carousel(number)
- Cycles the carousel to a particular item with at the given index.
Events
The carousel exposes the following events allowing the developer to tap into its behaviour.
- slide.carousel.responsive
-
This event is fired immediately when the plugins
slide
instance method is invoked. - slid.carousel.responsive
-
This event is fired when the plugins
slide
instance method is invoked once the carousel has completed sliding.
Data API
The carousel's default behaviour is bound using a markup API that allows running the plugin without writing a single line of JavaScript. If you need to override this behaviour the following code will allow you to do so.
// Override the default loading behaviour
$(window).off("load.carousel.responsive")
.on("load.carousel.responsive", function () {
// Your custom behaviour...
});
// Override the default carousel control click behaviour
$("YOUR_SELECTOR").off("click.carousel.responsive")
.on("click.carousel.responsive", "[data-carousel-slide]", function (event) {
// Your custom behaviour...
});
// Bind to the slide event.
$("YOUR_SELECTOR").on("slide.carousel.responsive", function(event) {
// Your custom behaviour...
});
// Bind to the slid event.
$("YOUR_SELECTOR").on("slid.carousel.responsive", function(event) {
// Your custom behaviour...
});